List Detail Page
In this tutorial, we will create a blog list and detail page from scratch.
Step 1: Content
Create a blog type and create two sample blog posts. You can refer to the content section for more details.
Step 2: View
Create a view to display the blog list. Here is the code for the component:
<script env="server">
var bloglist = k.content.blog.all();
</script>
<div k-for="item in bloglist">
<h2 k-content="item.title">Blog Title</h2>
<p k-content="item.summary">Blog Summary</p>
</div>
Step 3: Page
Create a blank page and place the component in it. Here is the code:
<html>
<body>
<view id="BlogList"></view>
</body>
</html>
You have made a blog list page.
If you want to create a page with a shared layout, you can use the following sample layout code:
<html>
<body>
<div k-placeholder="Main"> blog content </div>
</body>
</html>
When creating a page, you have the option to select a layout and configure the page by choosing relevant components, as shown in the following image.
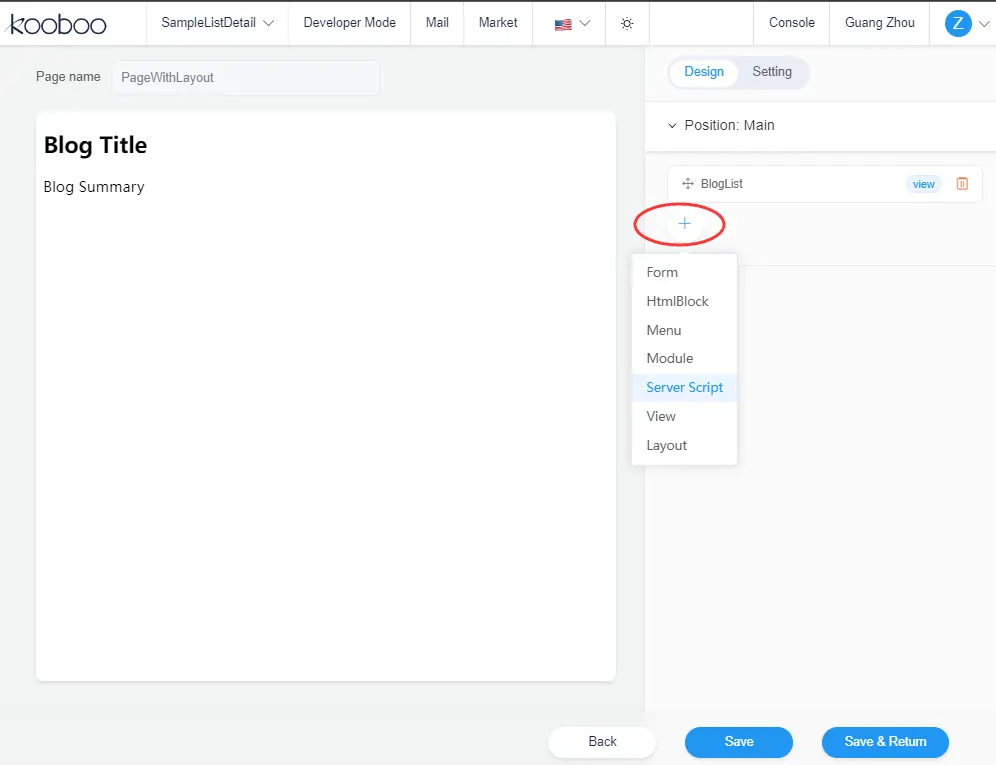
Step 4:Detail Page
Create a detail View that reads the details of a blog post. This page requires a parameter, specifically the UserKey from the content section. Here is an example code:
<script env="server">
var blog = k.content.blog.get(k.request.UserKey);
</script>
<div>
<h2 k-content="blog.title">Title</h2>
<p k-content="blog.detail">blog details</p>
</div>
Create a page and place the View in it, as shown in the code below:
<html>
<body>
<view id="BlogDetail"></view>
</body>
</html>
Step 5: Linking the List and Detail Pages
The detail page requires a parameter to know which blog post to read. In Kooboo, parameter passing is often automated, requiring no additional effort.
To achieve this, we simply add a link in the list View that points to the relevant detail page. With this, our blog list and detail page functionality will be complete.
The final list View is as follows:
<script env="server">
var bloglist = k.content.blog.all();
</script>
<div k-for="item in bloglist">
<h2 k-content="item.title">Blog Title</h2>
<p k-content="item.summary">Blog Summary</p>
<a href="/DetailPage"> Read More ...</a>
</div>
The generated HTML code for the page is as follows
<html>
<body>
<div>
<h2>blog title 1</h2>
<p>blog summary information 1</p>
<a href="/DetailPage?UserKey=blog-title-1"> Read More ...</a>
<h2>blog title 2</h2>
<p>blog summary information 2</p>
<a href="/DetailPage?UserKey=blog-title-2"> Read More ...</a>
</div>
</body>
</html>